Can Only Be Called on an Active Agent That Has Been Placed on a Navmesh.
Class AIPath Extends AIBase, IAstarAI
Public
AI for following paths.
This AI is the default motion script which comes with the A* Pathfinding Projection. It is in no fashion required by the rest of the system, so experience free to write your own. Merely I hope this script will make it easier to set up movement for the characters in your game. This script works well for many types of units, simply if you need the highest performance (for example if you lot are moving hundreds of characters) you lot may want to customize this script or write a custom movement script to be able to optimize it specifically for your game.
This script will endeavor to move to a given destination. At regular intervals, the path to the destination will be recalculated. If you desire to make the AI to follow a particular object you tin attach the AIDestinationSetter component. Take a await at the Go Started With The A* Pathfinding Project tutorial for more than instructions on how to configure this script.
Here is a video of this script being used movement an agent around (technically information technology uses the Pathfinding.Examples.MineBotAI script that inherits from this one but adds a flake of animation support for the example scenes):
Quick overview of the variables
In the inspector in Unity, you will see a agglomeration of variables. You tin view detailed information further down, simply here'southward a quick overview.
The repathRate determines how often it will search for new paths, if you take fast moving targets, you lot might want to set it to a lower value. The destination field is where the AI will attempt to move, it can be a indicate on the ground where the actor has clicked in an RTS for example. Or it can exist the player object in a zombie game. The maxSpeed is self-explanatory, equally is rotationSpeed. still slowdownDistance might require some explanation: It is the approximate altitude from the target where the AI will kickoff to slow down. Setting it to a large value will make the AI slow down very gradually. pickNextWaypointDist determines the altitude to the signal the AI will move to (encounter image beneath).
Below is an image illustrating several variables that are exposed by this class (pickNextWaypointDist, steeringTarget, desiredVelocity)
This script has many move fallbacks. If it finds an RVOController fastened to the aforementioned GameObject as this component, it will utilize that. If it finds a character controller it volition also utilise that. If it finds a rigidbody it will use that. Lastly it volition fall back to simply modifying Transform.position which is guaranteed to e'er piece of work and is also the nearly performant option.
How information technology works
In this section I'thousand going to become over how this script is structured and how data flows. This is useful if you want to make changes to this script or if you lot just desire to sympathise how it works a flake more deeply. However y'all do not need to read this section if you lot are just going to utilize the script as-is.
This script inherits from the AIBase grade. The movement happens either in Unity's standard Update or FixedUpdate method. They are both divers in the AIBase class. Which i is really used depends on if a rigidbody is used for movement or not. Rigidbody movement has to be done inside the FixedUpdate method while otherwise it is ameliorate to do information technology in Update.
From at that place a call is fabricated to the MovementUpdate method (which in turn calls MovementUpdateInternal). This method contains the main majority of the code and calculates how the AI *wants* to motion. However it doesn't do any movement itself. Instead it returns the position and rotation it wants the AI to movement to take at the end of the frame. The Update (or FixedUpdate) method then passes these values to the FinalizeMovement method which is responsible for actually moving the graphic symbol. That method likewise handles things like making sure the AI doesn't fall through the ground using raycasting.
The AI recalculates its path regularly. This happens in the Update method which checks shouldRecalculatePath and if that returns true information technology will call SearchPath. The SearchPath method will fix a path request and send it to the Seeker component which should be attached to the aforementioned GameObject as this script. Since this script will when waking up register to the Seeker.pathCallback delegate this script volition exist notified every time a new path is calculated past the OnPathComplete method beingness called. Information technology may accept one or sometimes multiple frames for the path to be calculated, simply finally the OnPathComplete method will be called and the current path that the AI is post-obit will be replaced.
Public Methods
void GetRemainingPath (
List<Vector3> | buffer | The buffer will be cleared and replaced with the path. The offset point is the current position of the agent. |
out bool | stale | May be true if the path is invalid in some way. For instance if the agent has no path or (for the RichAI script simply) if the amanuensis has detected that some nodes in the path take been destroyed. |
Fills buffer with the remaining path.
var buffer = new List<Vector3>();
ai.GetRemainingPath(buffer, out bool stale);
for (int i = 0; i < buffer.Count - i; i++) {
Debug.DrawLine(buffer[i], buffer[i+1], Color.crimson);
}
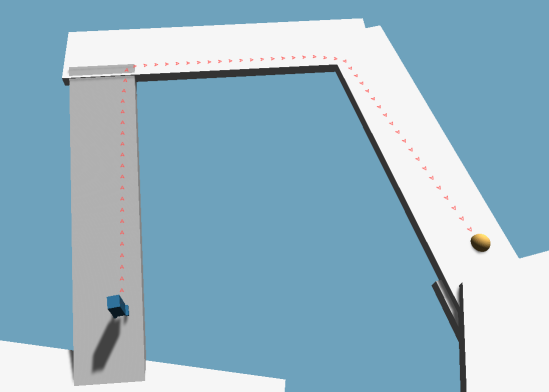
void OnTargetReached ( )
The end of the path has been reached.
If y'all want custom logic for when the AI has reached it's destination add information technology here. You can also create a new script which inherits from this i and override the role in that script.
This method volition be called again if a new path is calculated as the destination may have changed. So when the amanuensis is shut to the destination this method will typically be called every repathRate seconds.
void Teleport (
Vector3 | newPosition | |
bool | clearPath=true |
Instantly move the agent to a new position.
This volition trigger a path recalculation (if clearPath is true, which is the default) then if you desire to teleport the agent and modify its destination it is recommended that yous set the destination before calling this method.
The current path will be cleared by default.
See
Works similarly to Unity'due south NavmeshAgent.Warp.
SearchPath
Public Variables
bool alwaysDrawGizmos
Draws detailed gizmos constantly in the scene view instead of only when the amanuensis is selected and settings are being modified.
bool constrainInsideGraph = false
Ensure that the character is always on the traversable surface of the navmesh.
When this option is enabled a GetNearest query will exist done every frame to find the closest node that the amanuensis can walk on and if the agent is not inside that node, then the agent will be moved to it.
This is specially useful together with local abstention in order to avoid agents pushing each other into walls.
This option also integrates with local avoidance so that if the agent is say forced into a wall past other agents the local avoidance organisation will be informed about that wall and tin take that into account.
Enabling this has some functioning affect depending on the graph blazon (pretty fast for grid graphs, slightly slower for navmesh/recast graphs). If y'all are using a navmesh/recast graph you may want to switch to the RichAI movement script which is specifically written for navmesh/recast graphs and does this kind of clamping out of the box. In many cases it can likewise follow the path more smoothly around sharp bends in the path.
It is non recommended that you use this selection together with the funnel modifier on grid graphs considering the funnel modifier will brand the path get very close to the border of the graph and this script has a tendency to try to cut corners a bit. This may cause information technology to endeavor to go slightly exterior the traversable surface near corners and that will look bad if this option is enabled.
Warning
This option makes no sense to employ on indicate graphs because point graphs exercise not have a surface. Enabling this option when using a point graph will lead to the amanuensis being snapped to the closest node every frame which is likely not what you want.
Below you tin see an prototype where several agents using local avoidance were ordered to go to the same point in a corner. When not constraining the agents to the graph they are easily pushed inside obstacles.
float endReachedDistance = 0.2F
Altitude to the end bespeak to consider the terminate of path to be reached.
When the end is within this distance then OnTargetReached will exist called and reachedEndOfPath will return truthful.
bool hasPath
Truthful if this amanuensis currently has a path that it follows.
float maxAcceleration = -2.5f
How quickly the agent accelerates.
Positive values represent an dispatch in earth units per second squared. Negative values are interpreted as an inverse fourth dimension of how long it should have for the agent to accomplish its max speed. For example if it should take roughly 0.4 seconds for the agent to reach its max speed then this field should be ready to -one/0.iv = -2.v. For a negative value the final acceleration will be: -acceleration*maxSpeed. This behaviour exists more often than not for compatibility reasons.
In the Unity inspector there are two modes: Default and Custom. In the Default fashion this field is set to -2.5 which means that it takes about 0.4 seconds for the agent to achieve its top speed. In the Custom mode yous can set the acceleration to any positive value.
bool pathPending
Truthful if a path is currently existence calculated.
float pickNextWaypointDist = 2
How far the AI looks ahead along the path to make up one's mind the point it moves to.
In globe units. If you lot enable the alwaysDrawGizmos toggle this value will be visualized in the scene view as a blue circle effectually the agent.
Hither are a few example videos showing some typical outcomes with good values equally well as how it looks when this value is besides low and also loftier.
| Too low |
| Ok |
| Ok |
| Ok |
| As well high |
bool reachedDestination
Truthful if the ai has reached the destination.
This is a all-time effort calculation to see if the destination has been reached. For the AIPath/RichAI scripts, this is when the character is within endReachedDistance world units from the destination. For the AILerp script it is when the character is at the destination (±a very pocket-size margin).
This value will be updated immediately when the destination is changed (in contrast to reachedEndOfPath), however since path requests are asynchronous it volition utilize an approximation until information technology sees the existent path issue. What this holding does is to cheque the distance to the cease of the current path, and add to that the altitude from the cease of the path to the destination (i.e. is assumes it is possible to motility in a straight line between the finish of the current path to the destination) so checks if that total distance is less than endReachedDistance. This property is therefore only a best endeavor, but it will piece of work well for almost all utilize cases.
Furthermore it will not written report that the destination is reached if the destination is above the head of the grapheme or more than half the height of the character beneath its feet (so if you lot have a multilevel building, information technology is important that yous configure the height of the character correctly).
The cases which could be problematic are if an agent is standing side by side to a very sparse wall and the destination suddenly changes to the other side of that sparse wall. During the time that information technology takes for the path to be calculated the amanuensis may see itself every bit alredy having reached the destination because the destination simply moved a very pocket-sized altitude (the wall was sparse), even though it may actually be quite a long fashion effectually the wall to the other side.
In contrast to reachedEndOfPath, this holding is immediately updated when the destination is inverse.
IEnumerator Start () {
ai.destination = somePoint;
// Start to search for a path to the destination immediately
ai.SearchPath();
// Await until the agent has reached the destination
while (!ai.reachedDestination) {
yield return zippo;
}
// The amanuensis has reached the destination at present
}
bool reachedEndOfPath
Truthful if the amanuensis has reached the cease of the current path.
Note that setting the destination does non immediately update the path, nor is there any guarantee that the AI will actually be able to reach the destination that you set. The AI will try to go as shut as possible. Frequently you lot desire to apply reachedDestination instead which is easier to work with.
Information technology is very difficult to provide a method for detecting if the AI has reached the destination that works beyond all different games considering the destination may non even lie on the navmesh and how that is handled differs from game to game (see too the code snippet in the docs for destination).
bladder? remainingDistance
Approximate remaining distance along the current path to the end of the path.
The RichAI move script approximates this distance since it is quite expensive to calculate the real distance. However it will be authentic when the agent is within ane corner of the destination. You lot can utilize GetRemainingPath to summate the actual remaining path more precisely.
The AIPath and AILerp scripts utilise a more accurate distance calculation at all times.
If the agent does not currently take a path, so positive infinity will be returned.
Annotation
This is the distance to the stop of the path, which may or may not be at the destination. If the character cannot reach the destination information technology will try to move every bit close as possible to it.
Warning
Since path requests are asynchronous, there is a small delay between a path asking being sent and this value being updated with the new calculated path.
bladder rotationSpeed = 360
Rotation speed in degrees per second.
Rotation is calculated using Quaternion.RotateTowards. This variable represents the rotation speed in degrees per second. The college information technology is, the faster the character will be able to rotate.
bool slowWhenNotFacingTarget = true
Wearisome downwards when not facing the target direction.
Incurs at a small performance overhead.
float slowdownDistance = 0.6F
Distance from the end of the path where the AI will start to slow down.
Vector3? steeringTarget
Betoken on the path which the agent is currently moving towards.
This is ordinarily a bespeak a small altitude ahead of the amanuensis or the finish of the path.
If the agent does not have a path at the moment, then the agent's current position will be returned.
CloseToDestinationMode whenCloseToDestination = CloseToDestinationMode.Stop
What to do when within endReachedDistance units from the destination.
The character can either stop immediately when it comes within that distance, which is useful for eastward.g archers or other ranged units that want to fire on a target. Or the character can continue to try to reach the exact destination point and come to a full stop there. This is useful if you want the graphic symbol to reach the exact point that you specified.
Inherited Public Members
void FinalizeMovement (
Vector3 | nextPosition | New position of the agent. |
Quaternion | nextRotation | New rotation of the amanuensis. If enableRotation is fake so this parameter will be ignored. |
Moves the agent to a position.
This is used if you desire to override how the amanuensis moves. For example if you are using root move with Mecanim.
This will use a CharacterController, Rigidbody, Rigidbody2D or the Transform component depending on what options are available.
The amanuensis volition be clamped to the navmesh after the movement (if such data is bachelor, generally this is only done past the RichAI component).
void FindComponents ( )
Looks for any fastened components like RVOController and CharacterController etc.
This is done during OnEnable. If yous are adding/removing components during runtime you may want to telephone call this part to make sure that this script finds them. It is unfortunately prohibitive from a functioning standpoint to look for components every frame.
Vector3 GetFeetPosition ( )
Position of the base of the character.
This is used for pathfinding as the character'southward pin point is sometimes placed at the center of the grapheme instead of near the feet. In a edifice with multiple floors the center of a character may in some scenarios exist closer to the navmesh on the floor above than to the floor below which could cause an incorrect path to be calculated. To solve this the offset point of the requested paths is always at the base of the grapheme.
void Motility (
Vector3 | deltaPosition | Direction and altitude to move the agent in world space. |
Move the agent.
This is intended for external movement forces such as those applied by wind, conveyor belts, knockbacks etc.
Some movement scripts may ignore this completely (notably the AILerp script) if it does non have any concept of being moved externally.
The agent volition not be moved immediately when calling this method. Instead this offset will exist stored and and then practical the next time the agent runs its movement calculations (which is usually afterwards this frame or the next frame). If you want to motility the agent immediately then call: ai.Motility(someVector);
ai.FinalizeMovement(ai.position, ai.rotation);
void MovementUpdate (
float | deltaTime | time to simulate movement for. Ordinarily set to Fourth dimension.deltaTime. |
out Vector3 | nextPosition | the position that the amanuensis wants to move to during this frame. |
out Quaternion | nextRotation | the rotation that the amanuensis wants to rotate to during this frame. |
Calculate how the character wants to move during this frame.
Notation that this does not actually move the character. Yous need to call FinalizeMovement for that. This is called automatically unless canMove is false.
To handle movement yourself you tin can disable canMove and telephone call this method manually. This code volition replicate the normal behavior of the component: void Update () {
// Disable the AIs own movement code
ai.canMove = simulated;
Vector3 nextPosition;
Quaternion nextRotation;
// Calculate how the AI wants to move
ai.MovementUpdate(Fourth dimension.deltaTime, out nextPosition, out nextRotation);
// Modify nextPosition and nextRotation in whatever fashion you wish
// Really move the AI
ai.FinalizeMovement(nextPosition, nextRotation);
}
void SearchPath ( )
Recalculate the current path.
You lot can for case use this if you want very quick reaction times when you have changed the destination and then that the agent does not have to wait until the next automated path recalculation (encounter canSearch).
If there is an ongoing path adding, it will be canceled, then brand certain you get out time for the paths to get calculated before calling this office again. A canceled path volition show upwardly in the log with the message "Canceled by script" (see #Seeker.CancelCurrentPathRequest()).
If no destination has been set nonetheless then nix will be done.
Note
The path effect may not go bachelor until after a few frames. During the adding time the #pathPending holding will return truthful.
void SetPath ( )
Make the AI follow the specified path.
In case the path has not been calculated, the script will call seeker.StartPath to calculate it. This means the AI may not really start to follow the path until in a few frames when the path has been calculated. The #pathPending field will every bit usual return true while the path is being calculated.
In case the path has already been calculated it will immediately replace the current path the AI is post-obit. This is useful if you want to supplant how the AI calculates its paths. Annotation that if y'all calculate the path using seeker.StartPath then this script will already choice it upward because it is listening for all paths that the Seeker finishes calculating. In that instance you do not need to call this function.
If yous pass zippo every bit a parameter then the electric current path will be cleared and the agent volition stop moving. Note than unless you accept too disabled canSearch and then the agent will soon recalculate its path and start moving over again.
You lot tin can disable the automatic path recalculation by setting the canSearch field to false.
// Disable the automatic path recalculation
ai.canSearch = false;
var pointToAvoid = enemy.position;
// Make the AI flee from the enemy.
// The path will be near xx world units long (the default price of moving 1 world unit is grand).
var path = FleePath.Construct(ai.position, pointToAvoid, chiliad * 20);
ai.SetPath(path);
// If you want to brand use of backdrop like ai.reachedDestination or ai.remainingDistance or similar
// yous should also set the destination property to something reasonable.
// Since the agent'southward own path recalculation is disabled, setting this will not affect how the paths are calculated.
// ai.destination = ...
Colour ShapeGizmoColor = new Color(240/255f, 213/255f, 30/255f)
Quaternion SimulateRotationTowards (
Vector3 | direction | Direction in world space to rotate towards. |
float | maxDegrees | Maximum number of degrees to rotate this frame. |
Simulates rotating the agent towards the specified management and returns the new rotation.
Note that this but calculates a new rotation, information technology does not change the bodily rotation of the agent. Useful when you lot are handling movement externally using FinalizeMovement but you want to use the built-in rotation code.
AutoRepathPolicy autoRepath = new AutoRepathPolicy()
Determines how the agent recalculates its path automatically.
This corresponds to the settings under the "Recalculate Paths Automatically" field in the inspector.
bool canMove = true
Enables or disables movement completely.
If you lot desire the agent to stand even so, but nonetheless react to local abstention and utilize gravity: apply isStopped instead.
This is also useful if you lot want to have total control over when the movement calculations run. Take a look at MovementUpdate
Vector3? desiredVelocity
Velocity that this agent wants to move with.
Includes gravity and local avoidance if applicable.
Vector3 destination
Position in the earth that this agent should motion to.
If no destination has been set up yet, and so (+infinity, +infinity, +infinity) will exist returned.
Note that setting this holding does not immediately cause the agent to recalculate its path. And then it may accept some time before the agent starts to move towards this indicate. Virtually movement scripts have a repathRate field which indicates how oftentimes the agent looks for a new path. You can likewise phone call the SearchPath method to immediately showtime to search for a new path. Paths are calculated asynchronously and then when an agent starts to search for path it may have a few frames (ordinarily 1 or 2) until the result is available. During this time the #pathPending property will return true.
If you are setting a destination and so want to know when the agent has reached that destination so you could either use #reachedDestination (recommended) or check both #pathPending and #reachedEndOfPath. Cheque the documentation for the respective fields to learn nigh their differences.
IEnumerator Start () {
ai.destination = somePoint;
// Offset to search for a path to the destination immediately
ai.SearchPath();
// Wait until the agent has reached the destination
while (!ai.reachedDestination) {
yield render zip;
}
// The amanuensis has reached the destination at present
}
IEnumerator Showtime () {
ai.destination = somePoint;
// Start to search for a path to the destination immediately
// Note that the event may not get available until after a few frames
// ai.pathPending will be true while the path is beingness calculated
ai.SearchPath();
// Expect until we know for certain that the agent has calculated a path to the destination we set above
while (ai.pathPending || !ai.reachedEndOfPath) {
yield return cypher;
}
// The agent has reached the destination now
}
bool enableRotation = true
If true, the AI will rotate to confront the movement management.
Vector3 gravity = new Vector3(bladder.NaN, float.NaN, bladder.NaN)
Gravity to use.
If fix to (NaN,NaN,NaN) and so Physics.Gravity (configured in the Unity projection settings) will be used. If set to (0,0,0) then no gravity volition be used and no raycast to check for basis penetration volition be performed.
LayerMask groundMask = -i
Layer mask to utilize for ground placement.
Brand sure this does not include the layer of whatever colliders attached to this gameobject.
bladder height = 2
Tiptop of the amanuensis in earth units.
This is visualized in the scene view as a yellow cylinder around the grapheme.
This value is currently only used if an RVOController is fastened to the same GameObject, otherwise information technology is only used for cartoon overnice gizmos in the scene view. Yet since the meridian value is used for some things, the radius field is e'er visible for consistency and easier visualization of the character. That said, it may be used for something in a hereafter release.
Annotation
The Pathfinding.AILerp script doesn't really have any use of knowing the radius or the top of the character, so this property will always return 0 in that script.
bool isStopped
Gets or sets if the agent should cease moving.
If this is prepare to truthful the agent will immediately showtime to slow down as rapidly equally it can to come to a total end. The amanuensis will still react to local avoidance and gravity (if applicable), merely it will non try to move in whatever particular direction.
The current path of the agent will not be cleared, and so when this is set to imitation again the agent will continue moving along the previous path.
This is a purely user-controlled parameter, and then for instance information technology is not set automatically when the agent stops moving because it has reached the target. Use #reachedEndOfPath for that.
If this property is set up to true while the agent is traversing an off-mesh link (RichAI script only), then the amanuensis volition continue traversing the link and finish one time information technology has completed it.
Note
This is non the same as the canMove setting which some movement scripts take. The canMove setting disables movement calculations completely (which amid other things makes it not be afflicted by local avoidance or gravity). For the AILerp motility script which doesn't use gravity or local avoidance anyway changing this holding is very similar to changing canMove.
The #steeringTarget holding will continue to indicate the point which the agent would move towards if it would not be stopped.
float maxSpeed = 1
Max speed in world units per 2d.
IMovementPlane movementPlane = GraphTransform.identityTransform
Aeroplane which this agent is moving in.
This is used to convert between globe space and a motility plane to make information technology possible to utilize this script in both 2D games and 3D games.
System.Action onSearchPath
Chosen when the agent recalculates its path.
This is called both for automatic path recalculations (run across canSearch) and manual ones (meet SearchPath).
OrientationMode orientation = OrientationMode.ZAxisForward
Determines which direction the agent moves in.
For 3D games you most likely want the ZAxisIsForward option as that is the convention for 3D games. For 2D games you most likely want the YAxisIsForward selection equally that is the convention for 2D games.
Using the YAxisForward pick will also allow the agent to presume that the movement volition happen in the 2D (XY) plane instead of the XZ plane if it does non know. This is important but for the bespeak graph which does not have a well divers upward direction. The other congenital-in graphs (e.g the grid graph) will all tell the amanuensis which movement plane information technology is supposed to use.
Vector3? position
Position of the agent.
In globe infinite. If updatePosition is true then this value is idential to transform.position.
float radius = 0.5f
Radius of the amanuensis in earth units.
This is visualized in the scene view as a yellow cylinder around the character.
Annotation
The Pathfinding.AILerp script doesn't actually have any use of knowing the radius or the height of the character, so this holding will always return 0 in that script.
Quaternion? rotation
Rotation of the amanuensis.
If updateRotation is true and then this value is identical to transform.rotation.
bool updatePosition = true
Determines if the character's position should be coupled to the Transform'south position.
If fake then all motility calculations will happen as usual, merely the object that this component is attached to will not motion instead but the position belongings will modify.
This is useful if you want to control the movement of the graphic symbol using some other ways such as for instance root motion but still want the AI to move freely.
bool updateRotation = true
Determines if the character's rotation should be coupled to the Transform'southward rotation.
If fake so all movement calculations volition happen as usual, but the object that this component is attached to will not rotate instead only the rotation property will change.
Vector3? velocity
Actual velocity that the agent is moving with.
In world units per 2nd.
Private/Protected Members
void ApplyGravity ( )
Accelerates the amanuensis downwards.
void Awake ( )
Vector2 CalculateDeltaToMoveThisFrame (
Vector2 | position | |
bladder | distanceToEndOfPath | |
bladder | deltaTime |
Calculates how far to movement during a single frame.
void CalculateNextRotation ( )
void CalculatePathRequestEndpoints ( )
Outputs the start point and end betoken of the next automatic path asking.
This is a divide method to make it easy for subclasses to swap out the endpoints of path requests. For case the #LocalSpaceRichAI script which requires the endpoints to exist transformed to graph infinite commencement.
void CancelCurrentPathRequest ( )
void ClearPath ( )
Clears the electric current path of the agent.
Usually invoked using #SetPath(naught)
void FixedUpdate ( )
Called every physics update.
If rigidbodies are used then all movement happens hither.
Color GizmoColor = new Color(46.0f/255, 104.0f/255, 201.0f/255)
void MovementUpdateInternal ( )
Called during either Update or FixedUpdate depending on if rigidbodies are used for motility or not.
void OnDisable ( )
void OnDrawGizmos ( )
void OnDrawGizmosInternal ( )
void OnDrawGizmosSelected ( )
void OnEnable ( )
Called when the component is enabled.
void OnPathComplete ( )
Called when a requested path has been calculated.
A path is first requested by #UpdatePath, information technology is and then calculated, probably in the same or the next frame. Finally information technology is returned to the seeker which forwards it to this function.
int OnUpgradeSerializedData (
int | version | |
bool | unityThread |
Handle serialization backwards compatibility.
Vector3 RaycastPosition (
Vector3 | position | Position of the character in the world. |
float | lastElevation | Acme coordinate before the amanuensis was moved. This is forth the 'upwardly' axis of the movementPlane. |
Checks if the character is grounded and prevents ground penetration.
Sets verticalVelocity to cipher if the character is grounded.
Return
The new position of the character.
void Reset ( )
Handle serialization backwards compatibility.
Quaternion SimulateRotationTowards (
Vector2 | direction | Direction in the movement airplane to rotate towards. |
bladder | maxDegrees | Maximum number of degrees to rotate this frame. |
Simulates rotating the amanuensis towards the specified direction and returns the new rotation.
Note that this just calculates a new rotation, it does not change the actual rotation of the agent.
void Get-go ( )
Starts searching for paths.
If you override this method you should in most cases telephone call base of operations.Start () at the commencement of it.
void Update ( )
Chosen every frame.
If no rigidbodies are used then all motion happens here.
void UpdateVelocity ( )
bool IAstarAI. canMove
Enables or disables movement completely.
If you desire the amanuensis to stand nevertheless, just nonetheless react to local abstention and use gravity: use isStopped instead.
This is also useful if you want to take full command over when the movement calculations run. Take a await at MovementUpdate
bool IAstarAI. canSearch
Enables or disables recalculating the path at regular intervals.
Setting this to false does not cease whatsoever active path requests from existence calculated or stop it from continuing to follow the current path.
Annotation that this simply disables automated path recalculations. If you lot call the SearchPath() method a path will still exist calculated.
int gizmoHash = 0
float IAstarAI. elevation
Top of the agent in world units.
This is visualized in the scene view every bit a yellow cylinder effectually the graphic symbol.
This value is currently only used if an RVOController is attached to the same GameObject, otherwise it is only used for cartoon nice gizmos in the scene view. Even so since the height value is used for some things, the radius field is always visible for consistency and easier visualization of the character. That said, information technology may be used for something in a hereafter release.
Note
The Pathfinding.AILerp script doesn't really have any utilize of knowing the radius or the pinnacle of the grapheme, and so this property will always return 0 in that script.
float lastChangedTime = float.NegativeInfinity
Vector2 lastDeltaPosition
Amount which the graphic symbol wants or tried to move with during the last frame.
float lastDeltaTime
Delta time used for movement during the last frame.
float IAstarAI. maxSpeed
Max speed in world units per second.
Path path
Current path which is followed.
Vector3 prevPosition1
Position of the character at the stop of the last frame.
Vector3 prevPosition2
Position of the graphic symbol at the end of the frame before the final frame.
bladder IAstarAI. radius
Radius of the agent in world units.
This is visualized in the scene view equally a yellow cylinder effectually the character.
Note
The Pathfinding.AILerp script doesn't really have whatever use of knowing the radius or the superlative of the character, so this property will always return 0 in that script.
bool shouldRecalculatePath
Truthful if the path should be automatically recalculated as soon as possible.
Vector3 simulatedPosition
Position of the agent.
If updatePosition is true and then this value will exist synchronized every frame with Transform.position.
Quaternion simulatedRotation
Rotation of the agent.
If updateRotation is truthful and then this value volition be synchronized every frame with Transform.rotation.
bool usingGravity
Indicates if gravity is used during this frame.
Vector2 velocity2D
Current desired velocity of the agent (does not include local avoidance and physics).
Lies in the move aeroplane.
float verticalVelocity
Velocity due to gravity.
Perpendicular to the movement plane.
When the agent is grounded this may non accurately reflect the velocity of the agent. It may be non-zero even though the amanuensis is not moving.
bool waitingForPathCalculation = false
Only when the previous path has been calculated should the script consider searching for a new path.
Deprecated Members
Vector3 CalculateVelocity ( )
Current desired velocity of the agent (excluding physics and local abstention merely information technology includes gravity).
Deprecated
This method no longer calculates the velocity. Utilise the desiredVelocity property instead.
bool TargetReached
True if the finish of the path has been reached.
Deprecated
When unifying the interfaces for different movement scripts, this property has been renamed to reachedEndOfPath
float speed
Maximum speed in globe units per second.
Vector3 targetDirection
Direction that the amanuensis wants to motility in (excluding physics and local avoidance).
float turningSpeed
Rotation speed.
Deprecated
This field has been renamed to rotationSpeed and is now in degrees per 2d instead of a damping gene.
drinkardexpeconen.blogspot.com
Source: https://www.arongranberg.com/astar/docs/aipath.html
0 Response to "Can Only Be Called on an Active Agent That Has Been Placed on a Navmesh."
Post a Comment